
Let's Begin With Fibonacci Series In Java
To catch up with the Multibonacci code we must look up at the Fibonacci sequence code once and understand its logic.Here we will not use the general mathematical formula, though it makes the program shorter, instead we will divide logic in few statements for easy understanding which will help us climb the stairs to Multibonacci.
Here is the code to print first 10 members of Fibonacci sequence.
Source Code:
class fibonacci{ public static void main(String args[]) { int a=1,b=0,c=0; for(int i=1;i<=10; i++) { System.out.print(c+ ","); c=a+b; a=b; b=c; } } }
Output: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34
So, how does it works? First we take three int variables a,b and c, we are using a and b to store the previous two variables and c to take up there sum and print it. Then we change the values, a takes up the value of b, thus it gets the second last term, b gets the value of c thus it gets the last term, and thus it goes on. This is the logic that drives Multibonacci too.
The Multibonacci Series Concept
Once I came through an interesting program called “Tribonacci” series, where the last 3 members/terms had to be summed up to give the next term. So I thought there must be program to accommodate all these “Tribonacci”, “Tetrabonacci”, “Pentabonacci”……..and so on.Why Should I Learn It?
Multibonacci is not going to come for any exam or viva. But we should take up programming tasks up apart from the ‘syllabus’ to enhance our skills and understand the concepts well, which will make us enable not to just write programs we have learnt but say ‘Bring it on!!’ to programming challenges.Here Comes MULTIBONACCI Series In Java
As in Fibonacci we took two variables to store the previous two terms, in Multibonacci we are going to take an array whose length will be entered according to the user.
Source Code:
import java.io.*; class Multibonacci { public static void main (String args[])throws IOException { BufferedReader br=new BufferedReader(new InputStreamReader(System.in)); System.out.print("ENTER THE NUMBER OF MEMBERS TO BE ADDED UP: "); int x=Integer.parseInt(br.readLine()); int num[]=new int[x]; int printvar=0; //to store the sum and display int j,k; //looping variables. num[0]=1; System.out.print("Series: "); for (int m=1;m<x;m++) num[m]=0; for(int i=1;i<=10;i++) { System.out.print(printvar+ ", "); printvar=0; for(j=0;j<x;j++) printvar=printvar+num[j]; for (k=0;k<x-1;k++) num[k]=num[k+1]; num[x-1]=printvar; } } }
Output:
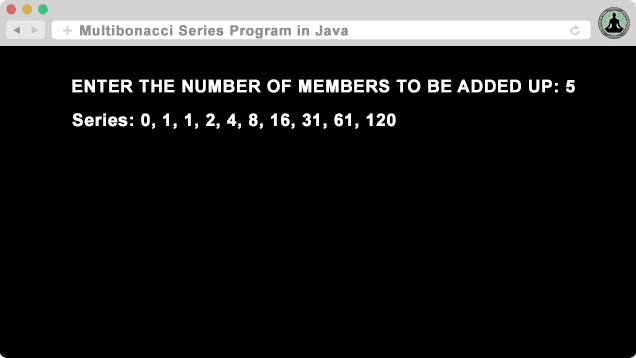
Conclusion: We can say that Multibonacci Series program is an advanced program of Fibonacci Series. We programmed this in Java but the concept is same everywhere, Multibonacci program is just for improvement of your programming skills, there's very less chance of coming this question in an interview, exam etc.
Hope you enjoyed this program... If you have any query or problem related to this program or any program, feel free to leave your comment below.
3 Comments Leave new
You might be interested in the following task from Rosetta code on the topic. It also includes the Lucas series. that start 2, 1, ...
ReplyYou might be interested in the following task from Rosetta code on the topic. It also includes the Lucas series. that start 2, 1, ...
http://rosettacode.org/wiki/Fibonacci_n-step_number_sequences
how to make a Inox ticket prg?
Replyfibonacci series pgm
ReplyMake sure you tick the "Notify Me" box below the comment form to be notified of follow up comments and replies.