Welcome to the second tutorial by Code Nirvana, here we are going to learn a very important aspect of Java programming, about data types and variables, there is virtually no good program you can write without the knowledge of these. From this tutorial we are going to use JDK 8, to keep ourselves updated. So let’s code on…
In case you missed our previous tutorial on Introduction to Java Programming, Visit Here
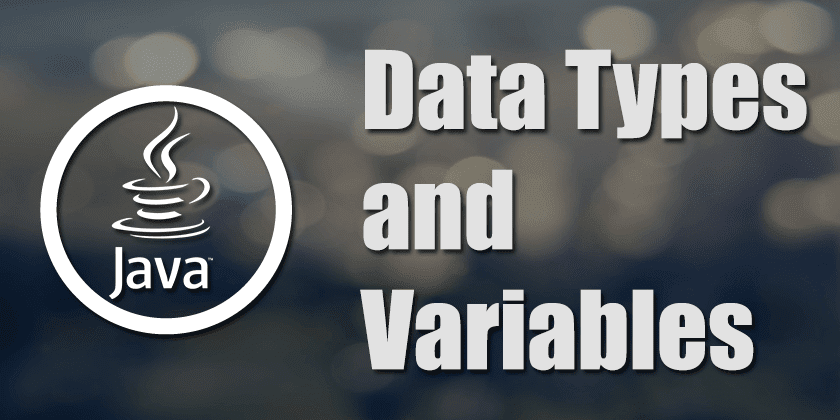
Variables in Java
Variables are simply named memory spaces to store some kind of data. Imagine you write up phone numbers of your class friends on separate papers and put it in separate envelopes and then you write the friend’s name on each envelope that you remember in which envelope you can find the required phone number. So, in this each friend’s name would be a variable and the paper and envelope would be the physical memory. A variable name should be meaningful and according to what it holds, this is a good practice but hard for lazy guys.. :)
But there are some restrictions on variable naming in Java, keep these in mind:
1. They must not begin with a digit.
2. Variable names are case sensitive. NIRVANA is not same as nirvana.
3. The name must not be a Java keyword.
4. You may give an underscore but white spaces…...banned!!!
Data Types in Java
Now, we know that variables hold data, but of what type? That information is specified by the variable’s data type. As Java has got distinct data types, it’s called a “Strongly typed language” unlike some other language like JavaScript.
Look at the hierarchy diagram to understand the organization of data types in Java.
In this tutorial we are going to learn about the left part of the diagram, i.e, Primitive data types.
INTEGER TYPES
Integer type of data type can hold whole number values, such as 1234, 656, -108..etc. There are four kinds of integer data types, which we use according to the size of value we need they are byte, short, int and long. They sizes that they can hold are like this:
TYPE
|
SIZE
|
VALUE RANGE
|
byte
|
1 byte
|
-128 to -127
|
short
|
2 bytes
|
-32,768 to 32,767
|
int
|
4 bytes
|
-2,147,483,648 to 2,147,483,647
|
long
|
8 bytes
|
-9,223,372,036,854,775,808 to
-9,223,372,036,854,775,807
|
CHOOSING THE CORRECT INTEGER TYPE
Now when we want to take a whole number in a variable which integer type to choose? As I said earlier it depends on the size of the constant/literal (value) it holds. Let’s try some example-
Suppose it’s a class roll no:-
short roll=12; [Don’t know how to declare a variable? Check previous tutorial]
Or, maybe it’s the cost of a laptop:-
int cost=23299; [In rupees]
Or, maybe it’s your friends phone number:-
Long number=1234567890;
Note: In common practice we use the int data type mostly for any size of variable, if it doesn't increase the size limit, then we use long.
FLOATIG POINT TYPES
Floating point types can hold decimal digits, it’s of two kinds float and double. Here also we choose the data type of the variable according to the size of the value. Actually float is ‘single-precision type’ and double is ‘double-precision type’, now in Java all the floating pint values are double-precision by default so when taking a value in float data type we must append a ‘f’ or ‘F’ after it.
Examples:
Suppose it’s a movie rating:
float rate=3.5F;
[If you don’t mention F a syntax error ‘possible loss of precision’ would show up]
Or, maybe it’s a precision length:
double length=19.2453;
Note: In common practice we mostly used double to avoid syntax errors.
The sizes of float and double are
TYPE
|
SIZE
|
RANGE
|
float
|
4 bytes
|
3.4e-038 to 1.7+0.38e
|
double
|
8 bytes
|
3.4e-038 to 1.7e+308
|
CHARACTER TYPE
In here we only have char data type; it’s used to store only one character. Don’t forget to enclose its value with single quotes(‘’)
Example:
char c=’a’;
BOOLEAN TYPES
They represent outcome of a condition, either true or false, these can be the only values of Boolean type. It is declared by boolean keyword.
Example:
boolean result=true;
Or,
boolean result=false;
There is also another String type, but we will discuss it when we will cover up classes, objects and functions. Because unlike the others String is a class, and we work with its objects.
TYPE CASTING IN JAVA
In Java we may sometimes need to change one type to another according our needs, and we may easily do this like this:
type2 variable2=(type2) variable1;
It will convert variable to type2.
Example:
int a=9;
double convert=(double)a;
The type is changed.
But we have to keep one thing in mind, some data types holds data of large sizes and some holds data of small sizes, so when we convert from a data type of large size to a data type of small size, there may be a loss of data. But the problem does not exist if it is vice versa.
Conclusion
So, this was it, we will discuss the String data type and scope of variables in some tutorials later. If you have any questions in this part please feel free to comment, don’t forget to subscribe the Code Nirvana newsletter or to share us on Facebook. To demand more tutorials, Contact Us. Keep Coding…
1 Comment. Leave new
Www.042tvseries.com
ReplyMake sure you tick the "Notify Me" box below the comment form to be notified of follow up comments and replies.